TOP 20 Coding Interview Question for Python
- Ammu Fredy
- Oct 19, 2022
- 2 min read
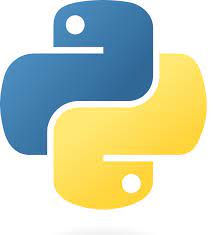
1: Convert a given string to int using a single line of code.
A = ‘5’
PRINT(INT(A))
5
2: Write a code snippet to convert a string to a list.
STR1 = "HI SUNSHINE"
PRINT(STR1.SPLIT(" "))
['HI', 'SUNSHINE']
3: Write a code snippet to reverse a string.
STR1 = “AMMU IS MY FRIEND”
STR2 = “ “
FOR I IN STR1
STR2= I+STR2
PRINT(STR2)
4: Write a code snippet to sort a list in Python.
X=[10,20,40,30]
PRINT(X.SORT())
5: What is the difference between mutable and immutable.
Mutable:it can’t be updated,list
Immutable:it can be updated ,tuple
6:How can you delete a file in Python.
IMPORT OS
OS.REMOVE(“TXT1.TXT”)
7: How to access an element of a list?
X=[1,2,3]
PRINT(X[0])
1
8: Discuss different ways of deleting an element from a list?
X=[1,2,3,4]
1.X.REMOVE(1)
2.X.POP(1)
9: Write a code snippet to delete an entire list?
X.CLEAR()
10: Write a code snippet to reverse an array.
IMPORT NUMPY AS NP
ARR1=NP.ARRAY([1,2,3,4])
ARR2=FLIP(ARR1)
PRINT(ARR1)
without function
IMPORT NUMPY AS NP
ARR1=NP.ARRAY([1,2,3,4])
ARR2=ARR1[::-1]
PRINT(ARR2)
11: Write a code snippet to get an element, delete an element and update an element in an array.
X=[1,2,3,4]
PRINT(X[0])#TO GET ELEMENT
X.REMOVE(2) #TO REMOVE ELEMENT
X.APPEND(6) # TO UPDATE THE ARRAY
12: How to concat two list
X=[1,2,3,4]
Y=[’W’,’Y’]
Z=X+Y
PRINT (Z)
Or
X=[1,2,3,4]
Y=[’W’,’Y’]
FOR I IN X
X.APPEND(Y)
PRINT X
or
LIST1 = ["A", "B" , "C"] LIST2 = [1, 2, 3] LIST1.EXTEND(LIST2) PRINT(LIST1)
13: To find the square of the list
X=[1,2,3,4]
V=[]
FOR I IN X :
V=I*2
PRINT (V)
14:what is shallow copy
A SHORTCUT WAY TO COPY A LIST OR A STRING IS TO USE THE SLICE OPERATOR [:] . THIS WILL MAKE A SHALLOW COPY OF THE ORIGINAL LIST KEEPING ALL OBJECT REFERENCES THE SAME IN THE COPIED LIST.
Z=[1,2,3,4]
X=Z
C=[:]
X[0]=1
C[5]=9
BOTH MEAN SHALLOW COPY,CHANGES MADE IN X,C WILL MAKE CHANGE INZ
15:What does :: 2 do in Python?
STRING[::2] READS “DEFAULT START INDEX, DEFAULT STOP INDEX, STEP SIZE IS TWO—TAKE EVERY SECOND ELEMENT”
16: What is the output, Suppose list1 is [1, 3, 2], What is list1 * 2?
[1, 3, 2, 1, 3, 2]
17: What is the output when we execute list(“hello”)?
[‘h’, ‘e’, ‘l’, ‘l’, ‘o’]
18:To find avg of a list
LIST1=[1,2,3]
SUM=SUM(LIST1)
PRINT(SUM)
LEN=LEN(LIST1)
AVERAGE=SUM/LEN
PRINT(LEN)
PRINT(AVERAGE)
19:How to reverse a number
N=23
A=STR(N)
REV = ' '
FOR I IN RANGE(0,LEN(A)):
REV = A[I] + REV
PRINT(REV)
20:How to reverse a word
N='HELLO'
REV = ' '
FOR I IN RANGE(0,LEN(N)):
REV = N[I] + REV
PRINT(REV)
21: How to reverse a string using slicer
N ="MY NAME IS AMMU"
C = []
B= N.SPLIT()[::-1]
FOR I IN B :
C.APPEND(I)
PRINT(" ".JOIN(C))
Comentários